JavaScript is a powerful and versatile programming language used in both frontend and backend development. As you dive deeper into JavaScript, you’ll come across concepts like closures. Closures are one of the more advanced concepts, but understanding them is crucial to becoming a proficient JavaScript developer.
Table of Contents
This comprehensive guide will help you understand what JavaScript closures are, how they work, and why they’re important for writing effective and efficient code.
What is a Closure in JavaScript?
A closure in JavaScript is created when a function is defined inside another function, allowing the inner function to access the variables of the outer function, even after the outer function has executed. Closures give the inner function access to its lexical scope, meaning the variables that were in scope at the time the function was created.
Definition:
In simpler terms, a closure is a combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). Closures allow you to remember the outer function’s variables, even after the function has returned.
Example of a Basic Closure:
function outerFunction() {
let outerVariable = "I am outside!";
function innerFunction() {
console.log(outerVariable); // Can access outerVariable even though outerFunction has completed execution
}
return innerFunction;
}
const myClosure = outerFunction();
myClosure(); // Logs: "I am outside!"
In this example, innerFunction
forms a closure, as it “remembers” the variable outerVariable
from the outerFunction
even after outerFunction
has finished executing.
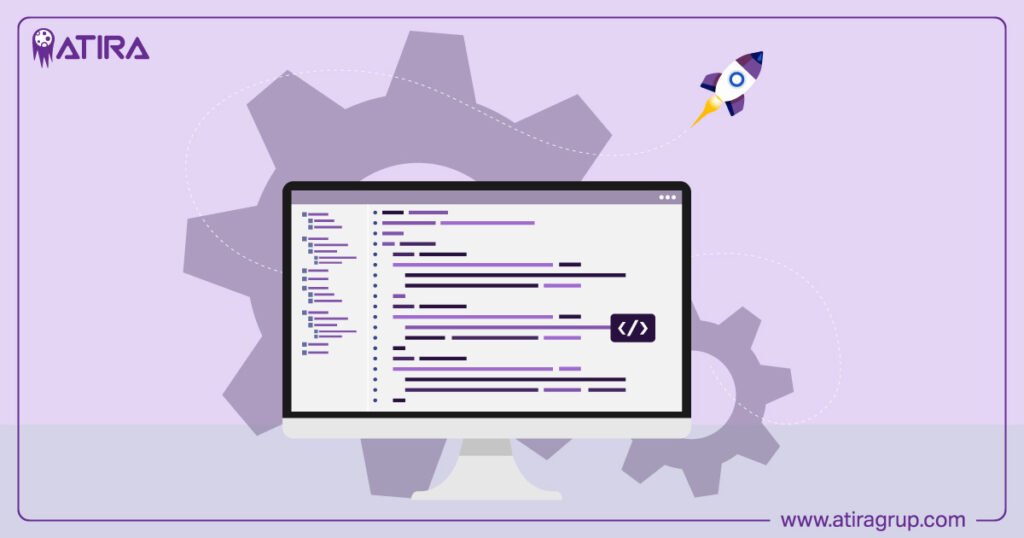
Understanding JavaScript Closures
How Closures Work
Closures work by preserving the lexical environment of the function. In JavaScript, when a function is created, a special link to the variables in its surrounding environment is also created. This link persists as long as the inner function exists, allowing it to access the variables even if the outer function is no longer active.
Key Points:
- A closure allows the inner function to access variables of the outer function, even after the outer function has returned.
- The inner function retains access to the scope chain of its lexical environment.
- Closures can be useful for data privacy, asynchronous operations, and callback functions.
Real-World Use Cases of Closures
Closures are not just a theoretical concept; they have many practical applications in everyday JavaScript development. Here are some common use cases:
1. Data Encapsulation (Private Variables)
Closures can be used to create private variables that cannot be accessed from outside the function, providing a layer of data protection.
function counter() {
let count = 0;
return function() {
count++;
console.log(count);
}
}
const increment = counter();
increment(); // 1
increment(); // 2
increment(); // 3
In this example, the count
variable is only accessible within the closure, preventing external code from modifying it directly. This is a common technique to encapsulate data and create private variables in JavaScript.
2. Callback Functions and Event Handlers
Closures are heavily used in event handlers and callback functions. Since the callback function often needs to access variables from its outer scope, closures allow this to happen seamlessly.
function buttonClickHandler(message) {
return function() {
alert(message);
}
}
const button1 = document.getElementById("button1");
button1.addEventListener("click", buttonClickHandler("Button 1 clicked!"));
const button2 = document.getElementById("button2");
button2.addEventListener("click", buttonClickHandler("Button 2 clicked!"));
Here, each button has its own closure, which allows the callback function to “remember” the specific message for each button click.
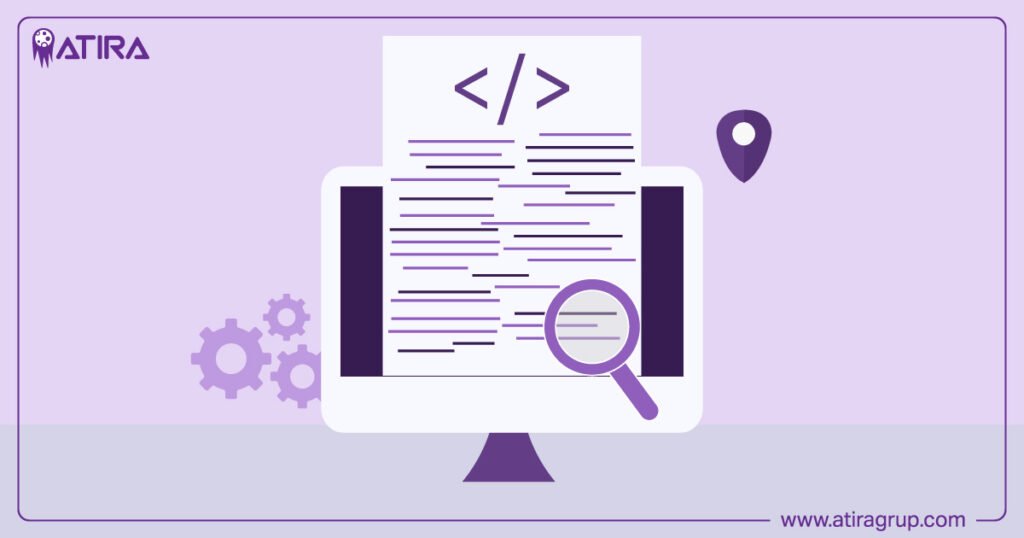
Understanding JavaScript Closures
3. Function Factories
You can use closures to create function factories—functions that generate other functions.
function createMultiplier(multiplier) {
return function(num) {
return num * multiplier;
}
}
const double = createMultiplier(2);
const triple = createMultiplier(3);
console.log(double(5)); // 10
console.log(triple(5)); // 15
In this example, createMultiplier
returns a function that remembers the value of multiplier
. This allows you to create different multiplier functions (e.g., double
and triple
) with specific behavior.
How Closures Affect Memory and Performance
While closures are powerful, they can also affect memory usage in JavaScript. Since closures hold references to variables in their outer scope, those variables are not automatically cleared from memory when the outer function returns. This can lead to memory leaks if closures are not managed properly.
When Closures Persist Memory:
Closures can hold onto more memory than expected, especially if they are used in long-running applications or within loops that create a large number of closures.
Best Practices for Managing Closures:
- Avoid unnecessary closures: If you don’t need to capture the outer variables, avoid creating closures, especially in loops or repetitive tasks.
- Use closures wisely: Limit the scope of closures to prevent unnecessary memory consumption.
- Garbage collection: Modern JavaScript engines like V8 (used in Chrome and Node.js) are efficient at garbage collection, but it’s still a good idea to be mindful of memory usage when dealing with closures.
Common Pitfalls of Closures
Closures are incredibly useful but can also introduce some unexpected behavior if not used correctly. Here are some common pitfalls to be aware of:
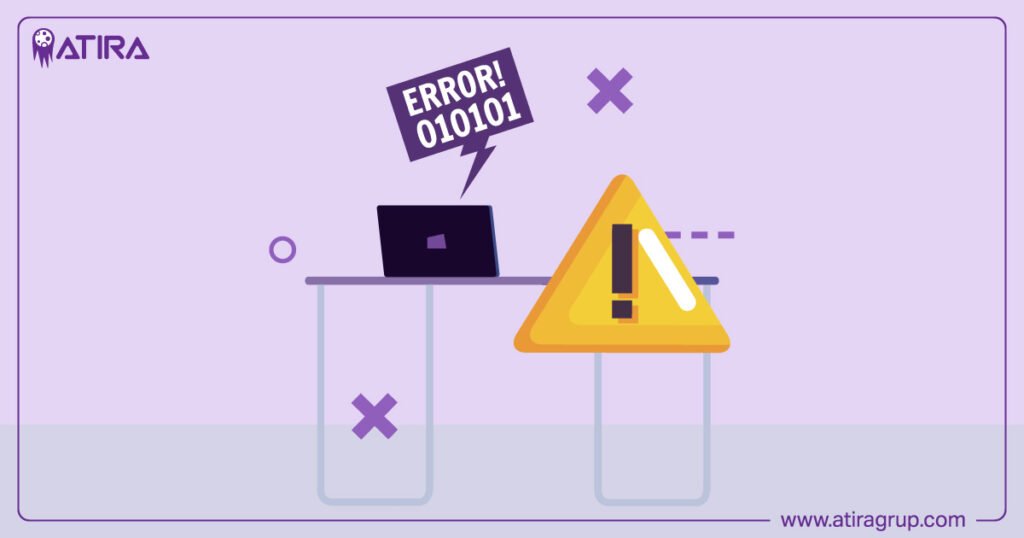
Understanding JavaScript Closures
1. Closures in Loops
One of the most common mistakes when using closures involves loops. If closures are created inside a loop without properly scoping the loop variable, the closure will reference the final value of the variable after the loop ends.
for (var i = 1; i <= 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
// After 1 second, logs: 4, 4, 4
This happens because the variable i
is scoped globally, so all the closures reference the final value of i
after the loop ends. To fix this, you can use let (which is block-scoped) instead of var or wrap the loop logic inside another function.
for (let i = 1; i <= 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
// Logs: 1, 2, 3
2. Unintended Memory Leaks
Since closures maintain references to their outer scope, they can inadvertently prevent memory from being freed, leading to memory leaks. This is especially problematic in large-scale applications where closures are used extensively.
3. Overuse of Closures
While closures are incredibly useful, overusing them can lead to complicated and hard-to-maintain code. For instance, using closures in scenarios where simpler solutions are available can make debugging more difficult and slow down the development process.
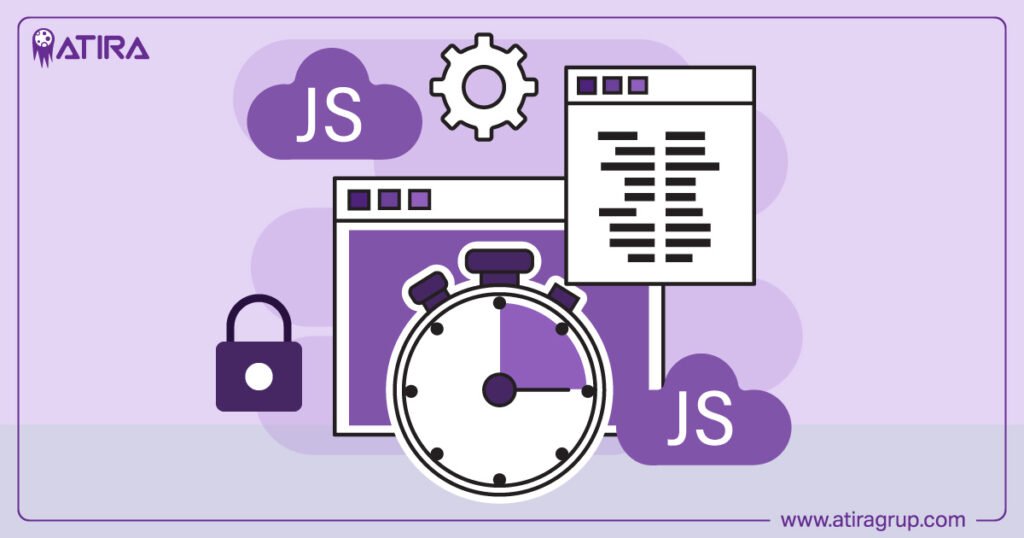
Understanding JavaScript Closures
Benefits of Closures
Closures offer a number of benefits in JavaScript development:
- Data Privacy: They allow you to create private variables that are not accessible from outside the function, providing better encapsulation.
- Maintain State: Closures enable you to maintain state across function calls, which is useful for scenarios like counters, caching, or configuration.
- Modularity: They help modularize code by allowing you to split functionality into smaller, reusable parts while maintaining access to external data.
- Asynchronous Programming: Closures play a crucial role in handling asynchronous tasks, such as callbacks, promises, and async/await.
Conclusion
Closures are an essential concept in JavaScript and play a significant role in the language’s functionality and flexibility. They allow functions to “remember” the variables of their outer scope, even after the outer function has returned, providing powerful ways to encapsulate data, handle asynchronous events, and maintain state.
Understanding closures is crucial for writing more efficient and modular code, making you a more skilled JavaScript developer. Whether you’re managing private variables, working with callbacks, or developing modular code, mastering closures will give you an edge in JavaScript development.
By now, you should have a solid grasp of what closures are, how they work, and how to use them effectively. The next step is to start experimenting with closures in your own projects and explore their many practical applications in web development.
Read More About:
Javascript as a Backend Language
Know more about JavaScript Closure